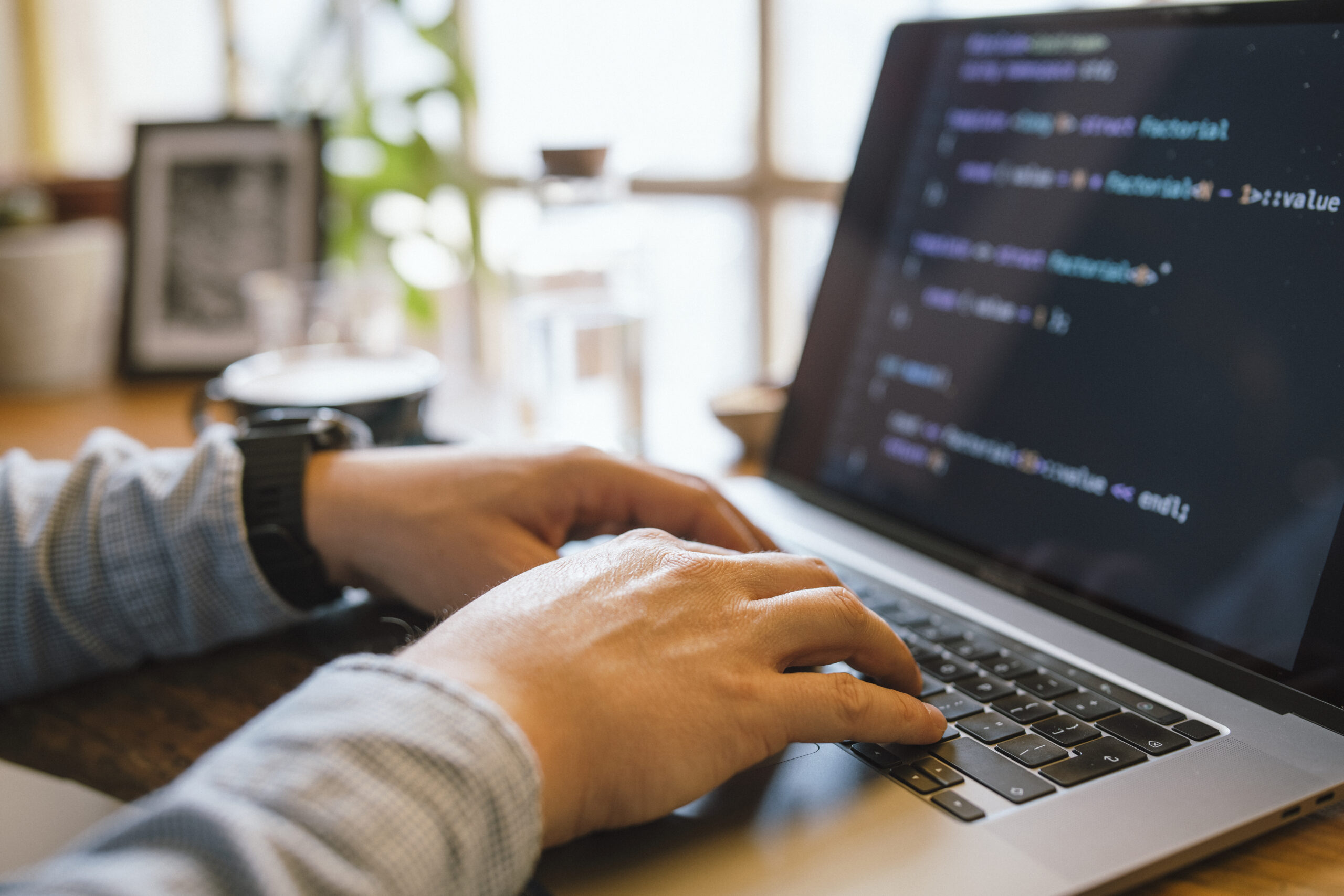
Debugging is The most necessary — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not almost repairing damaged code; it’s about knowledge how and why matters go Completely wrong, and learning to Consider methodically to resolve troubles successfully. No matter if you are a rookie or a seasoned developer, sharpening your debugging skills can save several hours of irritation and radically help your productivity. Listed here are a number of methods to help builders stage up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of several quickest ways builders can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though creating code is one Element of progress, recognizing tips on how to communicate with it effectively all through execution is Similarly essential. Contemporary development environments appear equipped with impressive debugging abilities — but numerous developers only scratch the floor of what these resources can perform.
Just take, for instance, an Built-in Advancement Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, phase by way of code line by line, as well as modify code within the fly. When utilized the right way, they Allow you to observe specifically how your code behaves throughout execution, which happens to be priceless for tracking down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclude developers. They help you inspect the DOM, keep track of community requests, see actual-time general performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch frustrating UI troubles into manageable jobs.
For backend or system-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Manage in excess of running processes and memory management. Mastering these tools might have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Variation control devices like Git to understand code history, locate the exact second bugs ended up introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely further than default configurations and shortcuts — it’s about building an intimate understanding of your growth natural environment making sure that when challenges crop up, you’re not misplaced at midnight. The better you realize your resources, the more time you can spend fixing the actual problem rather than fumbling through the process.
Reproduce the condition
One of the more crucial — and often missed — ways in productive debugging is reproducing the challenge. Ahead of leaping to the code or producing guesses, developers have to have to make a steady atmosphere or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a video game of possibility, frequently resulting in squandered time and fragile code improvements.
Step one in reproducing a problem is collecting as much context as feasible. Talk to issues like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or error messages? The greater detail you have got, the less difficult it becomes to isolate the precise conditions under which the bug happens.
Once you’ve gathered enough facts, attempt to recreate the situation in your neighborhood environment. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking method states. If the issue appears intermittently, look at writing automated checks that replicate the edge situations or state transitions concerned. These checks not only support expose the condition but additionally protect against regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on certain functioning systems, browsers, or below distinct configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. With a reproducible scenario, You should use your debugging resources much more efficiently, examination prospective fixes securely, and converse additional Plainly with the staff or end users. It turns an summary grievance into a concrete problem — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Error messages in many cases are the most worthy clues a developer has when anything goes Mistaken. As an alternative to viewing them as irritating interruptions, developers ought to learn to take care of mistake messages as direct communications from the procedure. They generally inform you just what exactly took place, exactly where it happened, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the information meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, glance at the 1st line and quickly begin earning assumptions. But deeper in the mistake stack or logs may lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them initial.
Crack the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can greatly quicken your debugging process.
Some mistakes are obscure or generic, As well as in These situations, it’s very important to examine the context during which the mistake occurred. Check out linked log entries, input values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These generally precede larger problems and provide hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them the right way turns chaos into clarity, helping you pinpoint problems more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an software behaves, assisting you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with realizing what to log and at what degree. Typical logging levels include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic data in the course of advancement, Information for general situations (like thriving start out-ups), WARN for possible troubles that don’t break the applying, Mistake for real issues, and Lethal if the program can’t continue.
Steer clear of flooding your logs with excessive or irrelevant facts. Excessive logging can obscure essential messages and decelerate your technique. Give attention to key gatherings, condition adjustments, input/output values, and significant conclusion factors in your code.
Structure your log messages clearly and continuously. Contain context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments exactly where stepping by code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-assumed-out logging method, it is possible to lessen the time it will take to spot difficulties, acquire deeper visibility into your apps, and Increase the All round maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently recognize and correct bugs, builders will have to approach the process just like a detective fixing a thriller. This way of thinking allows break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, gather as much related data as you may devoid of leaping to conclusions. Use logs, examination situations, and consumer reviews to piece with each other a clear picture of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What can be producing this habits? Have any improvements just lately been manufactured for the codebase? Has this situation occurred before less than very similar situation? The aim would be to slender down options and discover likely culprits.
Then, examination your theories systematically. Attempt to recreate the condition in a very controlled environment. When you suspect a particular function or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Pay out shut consideration to modest particulars. Bugs normally conceal in the the very least anticipated sites—just like a lacking semicolon, an off-by-a single mistake, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty without having fully comprehension it. Temporary fixes may possibly hide the true issue, just for it to resurface later.
And finally, continue to keep notes on Everything you tried using and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming difficulties and help here Other folks understand your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering hidden concerns in intricate units.
Write Exams
Composing assessments is among the simplest methods to boost your debugging techniques and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-confidence when building variations towards your codebase. A well-tested application is easier to debug because it enables you to pinpoint specifically the place and when a difficulty happens.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily reveal regardless of whether a particular piece of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to look, noticeably lessening enough time put in debugging. Unit tests are Particularly helpful for catching regression bugs—issues that reappear just after Earlier getting set.
Next, combine integration exams and finish-to-close assessments into your workflow. These aid be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various factors or providers interacting. If something breaks, your assessments can tell you which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Consider critically about your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code composition and fewer bugs.
When debugging a concern, creating a failing exam that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to center on fixing the bug and observe your take a look at pass when the issue is fixed. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable system—helping you capture extra bugs, faster and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Alternative after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and often see the issue from the new standpoint.
If you're as well close to the code for as well lengthy, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be considerably less productive at difficulty-solving. A short wander, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report finding the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display screen, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re trapped, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may experience counterintuitive, Specifically less than restricted deadlines, but it really truly causes quicker and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weak spot—it’s a smart tactic. It gives your Mind House to breathe, improves your point of view, and allows you avoid the tunnel eyesight That always blocks your development. Debugging is actually a psychological puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you face is a lot more than just A brief setback—It is really an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural concern, each can instruct you a little something beneficial should you make the effort to replicate and review what went Improper.
Start off by inquiring on your own a handful of key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically expose blind places in the workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing That which you've realized from a bug with all your friends could be Particularly impressive. Irrespective of whether it’s by way of a Slack message, a brief generate-up, or a quick understanding-sharing session, encouraging Some others stay away from the same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In any case, some of the finest developers will not be the ones who publish perfect code, but people that continuously understand from their mistakes.
In the long run, each bug you correct provides a fresh layer towards your skill set. So future time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging competencies requires time, follow, and tolerance — however the payoff is big. It would make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.